Web Components: An Overview
Web Components consist of standardized APIs that enable developers to create custom, reusable HTML tags. These tags encapsulate both functionality and styles away from the global scope, offering significant benefits in terms of code management. The core technologies that make up Web Components include Custom Elements, the Shadow DOM, and ES Modules.
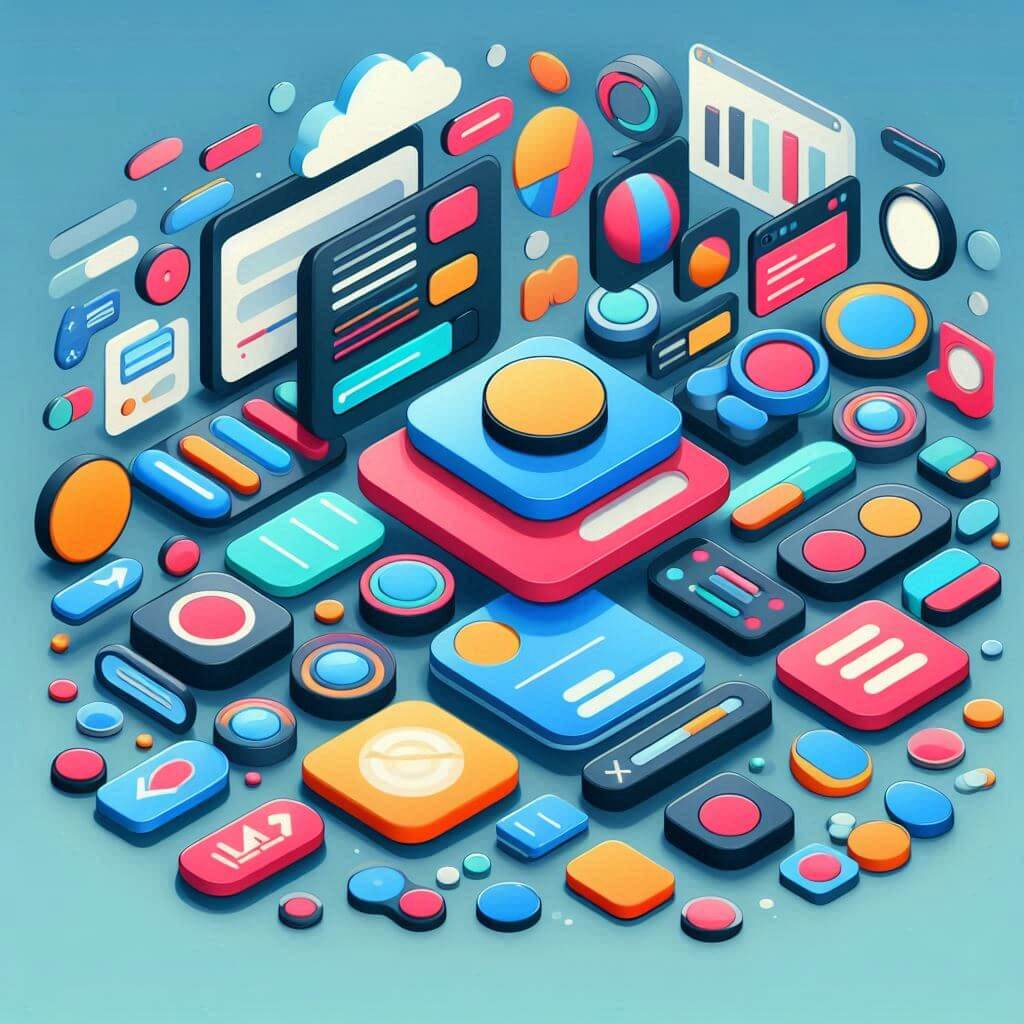
Custom Elements allow developers to define new HTML elements along with their behavior. These elements can be extended from existing HTML elements or created from scratch. The Shadow DOM offers encapsulated styling and DOM hierarchy, enabling developers to isolate parts of their code and avoid conflicts. ES Modules are employed to define modules in JavaScript, simplifying dependency management.
Custom Elements: Empowering Reusability
Custom elements are key to Web Components, providing a method to define new HTML tags that encapsulate the behavior and rendering of a component. They are pivotal in enhancing reusability, allowing developers to use the same components across multiple pages or applications without redundancy. The maintenance process becomes more straightforward as updating a component once propagates changes throughout its usage. Furthermore, custom elements are framework-agnostic, meaning they can be utilized with any frontend framework, such as React, Vue, Angular, or Vanilla JavaScript.
Creating a custom element involves extending the `HTMLElement` class and defining the component’s functionalities. For example:
“`javascript
class MyCustomElement extends HTMLElement {
constructor() {
super();
this.attachShadow({ mode: ‘open’ });
}
connectedCallback() {
this.shadowRoot.innerHTML = `<p>Hello world!</p>`;
}
}
customElements.define(‘my-custom-element’, MyCustomElement);
“`
The example above demonstrates the creation of a custom element that displays “Hello world!” upon being added to the DOM.
Shadow DOM: Stronger Encapsulation
The Shadow DOM provides robust encapsulation by isolating a component’s internal structure and style from the main document’s DOM tree. This encapsulation is crucial for several reasons, including style and script isolation, CSS scoping, and DOM isolation. Styles and scripts defined within a Shadow DOM do not affect the main document and vice versa, ensuring that the styles are scoped to the component and preventing conflicts.
Implementing the Shadow DOM in a custom element, as shown below, helps achieve encapsulated styling:
“`javascript
class ShadowComponent extends HTMLElement {
constructor() {
super();
this.attachShadow({ mode: ‘open’ });
}
connectedCallback() {
this.shadowRoot.innerHTML = `
<style>
p {
color: green;
}
</style>
<p>This is a shadow dom paragraph!</p>
`;
}
}
customElements.define(‘shadow-component’, ShadowComponent);
“`
The paragraph within the Shadow DOM has isolated styling, with the text colored green, unaffected by global styles.
Benefits of Web Components and Shadow DOM
Adopting Web Components and Shadow DOM offers numerous benefits. Reusable UI components save development time and effort by reducing code redundancy. Maintaining a single source of truth for each component also ensures efficient application-wide changes. The compatibility of Web Components across various frontend frameworks allows teams to select the best tools for each task without being locked into a specific ecosystem. Shadow DOM ensures proper encapsulation, preventing global stylesheet or JavaScript interference, which is particularly beneficial in large-scale applications where conflicts might arise. Encapsulated components are also easier to test independently, leading to more reliable and maintainable code.
Future Implications for Web Development
One notable future implication is the potential for standardization in how UI components are developed and shared across projects. As Web Components are built on standardized web technologies, they ensure that components adhere to universal guidelines, making them easier to integrate and maintain. This standardization fosters consistency, leading to smoother collaboration between teams and reducing the friction caused by disparate coding practices.
With built-in support from modern browsers, Web Components are optimized for performance. Since Web Components are native to the web platform, there’s no need for additional abstraction layers or complex frameworks, which can slow down application performance. The ability to encapsulate styles and functionality using the Shadow DOM means that components are loaded and rendered more efficiently. Faster load times and better performance are particularly critical for mobile applications and Progressive Web Apps (PWAs), where every millisecond counts in delivering a smooth user experience.
The encapsulation provided by the Shadow DOM ensures that only the necessary styles and scripts are applied to specific components, reducing the browser’s rendering workload and avoiding common pitfalls like global CSS issues.
Web Components’ modular nature contributes to enhanced scalability in modern web applications. Large-scale applications can be broken down into smaller, manageable components, each handling a specific piece of functionality. This modular approach simplifies the development process, as different teams can work on separate components without worrying about integration conflicts.
Changes or updates to one component do not necessarily impact others, making it easier to scale applications as new features are added or existing ones are modified. As the application grows, the modular structure allows for more straightforward debugging, maintenance, and refactoring.
The growth of an ecosystem around Web Components is another significant future implication. As more developers and organizations see the benefits of using Web Components, the number of open-source libraries and tools will continue to increase. This expanding ecosystem provides a wide range of pre-built, well-documented, and tested components that developers can readily incorporate into their projects.
For instance, platforms like GitHub and npm already host numerous Web Component libraries that offer solutions for common UI patterns and functionalities. The community-driven development ensures rapid innovation, peer review, and continuous improvement, enhancing the quality and reliability of available components.
As Web Components gain traction, tools and frameworks will evolve to provide enhanced support, simplifying their adoption and integration. Development environments, debugging tools, and testing frameworks will likely introduce features specifically tailored for Web Components and the Shadow DOM.
Best Practices for Using Web Components and Shadow DOM
Maximizing the advantages of Web Components and Shadow DOM requires adherence to best practices like designing modular components that encapsulate specific functionality. While Shadow DOM provides great encapsulation, it should be used judiciously to avoid over-isolation; sometimes global styles can suffice. Leveraging existing Web Component libraries can save development time and promote consistency. Staying updated with the latest developments and best practices ensures your implementation remains current, and prioritizing accessibility ensures your components are usable by a wider audience.
Tools and Libraries Supporting Web Components and Shadow DOM
Several tools and libraries support the development of Web Components and Shadow DOM. Lit offers a simple way to build Web Components using the native web platform. Stencil serves as a compiler generating Web Components, enabling the creation of fast, reusable components. Storybook, while not exclusive to Web Components, is an excellent tool for developing and testing individual components in isolation.
Web Components and Shadow DOM signify a significant shift in frontend development. By embracing these technologies, developers can create more maintainable, reusable, and interoperable components. As the ecosystem continues to evolve, these innovations will likely become integral to modern web development strategies.